I was able to get a shadow effect in Xamarin Forms for a box view, I'm pretty sure it can used similarly for a Frame. I got the clue from Android Documentation
I added a new property called HasShadow
public static readonly BindableProperty HasShadowProperty =
BindableProperty.Create("HasShadow", typeof(bool), typeof(ExtendedBoxView), false);
public bool HasShadow
{
get { return (bool)GetValue(HasShadowProperty); }
set { SetValue(HasShadowProperty, value); }
}
Here's the code for the Renderer in Android
public class ExtendedBoxViewRenderer : BoxRenderer
{
protected override void OnElementChanged(ElementChangedEventArgs<BoxView> e)
{
base.OnElementChanged(e);
var element = e.NewElement as ExtendedBoxView;
if (element == null) return;
if (element.HasShadow)
{
ViewGroup.Elevation = 8.0f;
ViewGroup.TranslationZ = 10.0f;
}
}
}
And this is how it looks
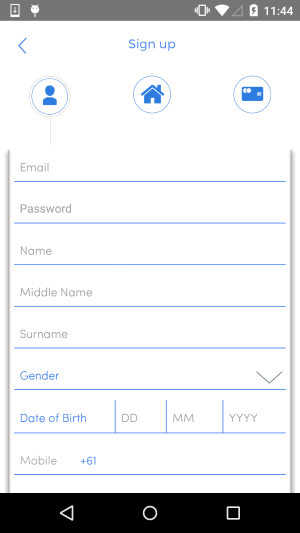
UPDATE
I found out that this approach causes app crash for older versions of Android. Although I haven't found a way to display Shadows in Android versions prior to Lollipop. This will prevent any app crashes
public class ExtendedBoxViewRenderer : BoxRenderer
{
protected override void OnElementChanged(ElementChangedEventArgs<BoxView> e)
{
base.OnElementChanged(e);
var element = e.NewElement as ExtendedBoxView;
if (element == null) return;
if (element.HasShadow)
{
//For some reason ViewCompat has issues when running in debug hence the workaround.
#if DEBUG
double dAndroidVersion;
if (double.TryParse(Build.VERSION.Release, out dAndroidVersion))
{
if (dAndroidVersion < 21)
return;
}
#else
ViewCompat.SetElevation(ViewGroup, 8.0f);
ViewCompat.SetTranslationZ(ViewGroup, 10.0f);
#endif
}
}
}