Here is Mike's awesome code put into a reusable function:
function reverseCssCubicBezier(cubicBezier) {
var maxX = Math.max(cubicBezier[0].x, cubicBezier[1].x, cubicBezier[2].x, cubicBezier[3].x);
var maxY = Math.max(cubicBezier[0].y, cubicBezier[1].y, cubicBezier[2].y, cubicBezier[3].y);
var halfUnitTurn = function(v) {
var tx = maxX/2, ty = maxY/2;
return { x: tx - (v.x-tx), y: ty - (v.y-ty) };
};
var revd = cubicBezier.map(halfUnitTurn);
return revd.reverse();
}
And this is how to use it:
var ease = [{x:0,y:0}, {x:.25,y:.1}, {x:.25,y:1}, {x:1,y:1}]; //cubic-bezier(.25, .1, .25, 1)
var ease_reversed = reverseCssCubicBezier(ease);
var ease_css_string = 'cubic_bezier(' + [ease[1].x, ease[1].y, ease[2].x, ease[2].y].join(', ') + ')';
var ease_reversed_css_string = 'cubic_bezier(' + [ease_reversed[1].x, ease_reversed[1].y, ease_reversed[2].x, ease_reversed[2].y].join(', ') + ')';
This returns:
ease: [{x:0, y:0}, {x:0.25, y:0.1}, {x:0.25, y:1}, {x:1, y:1}]
ease-reversed: [{x:0, y:0}, {x:0.75, y:0}, {x:0.75, y:0.9}, {x:1, y:1}]
ease: cubic_bezier(0.25, 0.1, 0.25, 1)
ease-reversed: cubic_bezier(0.75, 0, 0.75, 0.9)
Graphically we see its perfect overlap, i shifted the green one to left a little bit to show its perfectly overlapping.
Thanks Mike!
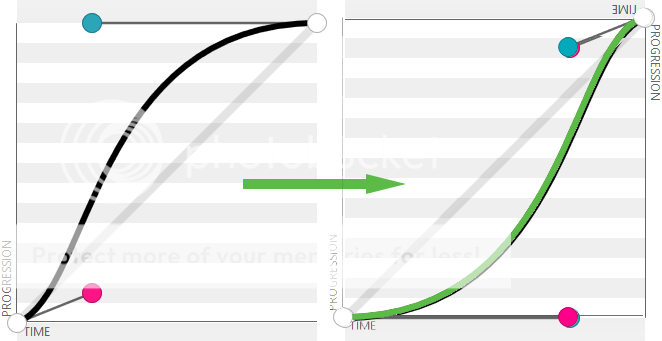